Boids, the abbreviation of "Bird-like objects," caught my attention online when I saw these amazing simulations of large schools of fish as a part of a GDC talk for the game "Abzu." This led to me researching and documenting how to create these Boids on my own as a part of a personal Open-Ended research project. Everything below is a simplified version of what this project entailed, but I recommend you look at my GitHub if you're interested in the finer details.
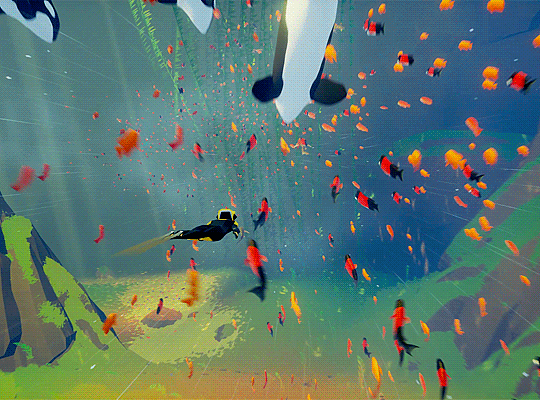
An example of using Boids in the game Abzu to simulate schools of fish.
What are Boids? Boids are an advanced particle system where individual "particles" (simulated birds) navigate based on local perception, simulated physics, and predefined behaviors. Originating from Craig W. Reynolds's model, Boids interact dynamically with each other, shaping their motion and orientation based on both internal states and external environmental factors. This enables realistic, fluid movement, with each "boid" adjusting within a flexible, flying coordinate system.
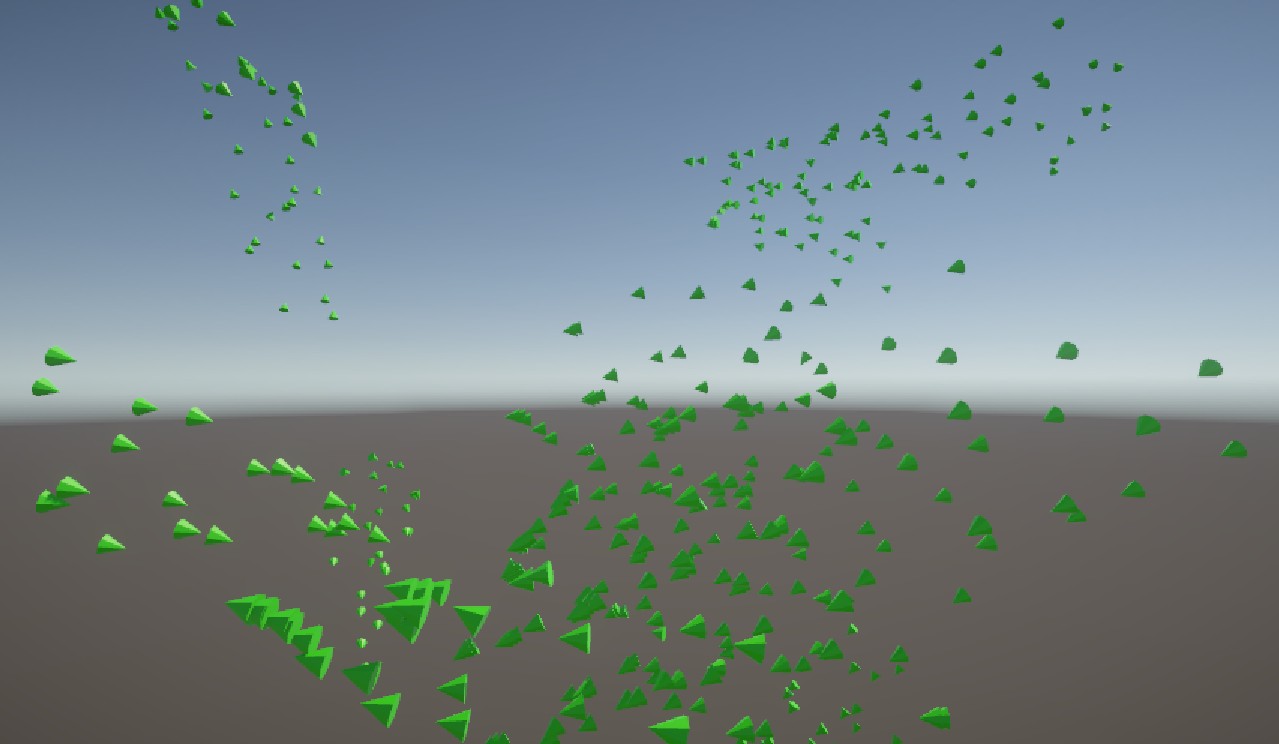
A screenshot taken from me simulating 250 Boids running simultaneously in an open space
When implementing these Boids, we must understand the rules an individual Boid follows. Boid behavior in simulated flocking follows three main rules: Collision Avoidance (avoiding others), Velocity Matching (aligning with others), and Flock Centering (staying near others), often simplified as Separation, Alignment, and Cohesion. When implemented, these rules create a basic but adaptive Boid capable of responding dynamically to its surroundings. However, the computational demands increase significantly with larger flocks, as each Boid requires continuous frame-by-frame calculations to check for changes and avoid obstacles, highlighting the need to optimize for scalability.
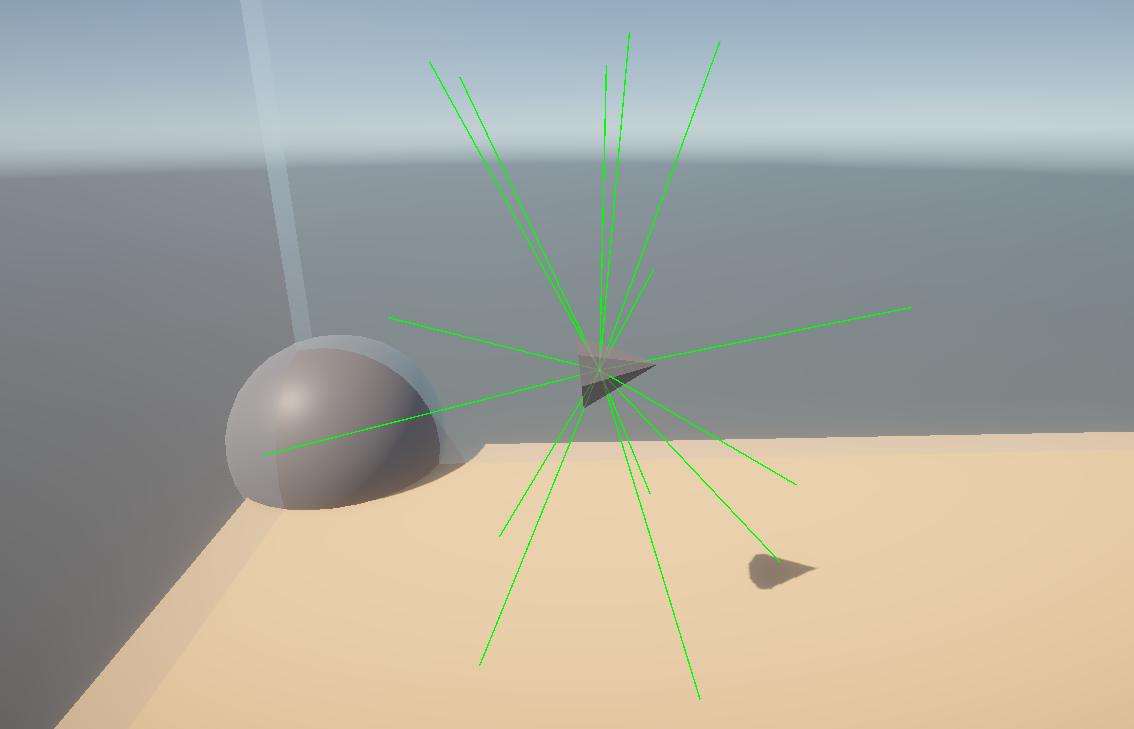
Here, we visualize the raycasts being sent out by an individual Boid. These raycasts allow the Boid to perceive nearby obstacles and to adapt that Boid's local path.
While the current Boids implementation effectively demonstrates flocking behavior, performance and pathfinding improvements could make it more robust. Currently, a large number of active Boids (over 100) can lead to FPS drops due to CPU bottlenecks from constant frame-by-frame updates. Utilizing compute shaders on the GPU, as suggested by Sebastian Lague, could alleviate this, though this requires expertise in DirectX 11 HLSL, which I’m still learning.
The current method of pathfinding uses spherical raycasts to avoid obstacles. A potential enhancement is to limit the detection area to a forward cone, mimicking the boid’s “field of vision” and allowing for more realistic navigation. Additionally, dynamically adjusting the boid’s speed and turn speed when obstacles are detected could prevent clipping issues and enable smoother, more adaptive movement.
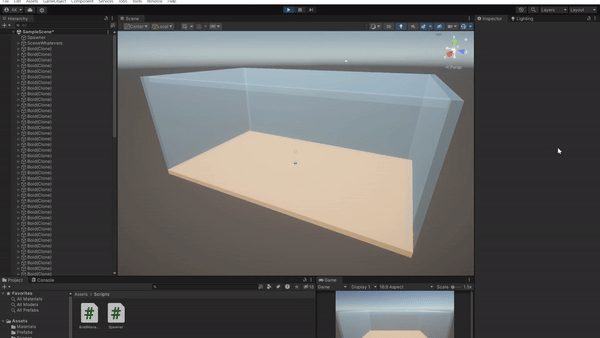
An Early clip of a hundred Boids moving with no Obstacles obscuring their path.
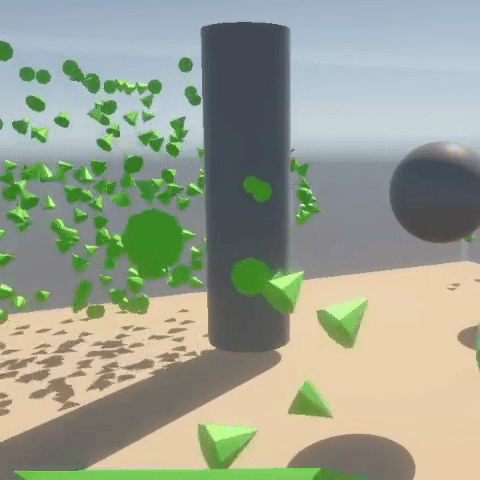
Finalized working Boids.
This research process was both educational and enjoyable, as I applied what I learned to implement Boids in a functional system. My GitHub outlines several potential improvements to my current Boids implementation, particularly regarding performance. At the time, I hadn’t yet developed a deep enough understanding of compute shaders on the GPU to address these issues, but that would be my priority if I were to revisit the project. Overall, this experience was a valuable opportunity to bring a theoretical concept to life in C#, and I’m pleased with the outcome.